Sprint 1: Project Setup and Planning (Estimated Time: 2-3 days)
In this sprint, we will set up the project and plan the overall architecture of the application.
Tasks:
- Create a new ASP.NET Core project:
- Open Visual Studio and create a new project.
- Choose "ASP.NET Core Web Application" and select the desired template (e.g., Web API, MVC, etc.).
- Name the project and choose a location.
- Choose the project architecture:
- Decide on the project architecture (e.g., monolithic, microservices, etc.).
- Determine the required layers (e.g., data access, business logic, presentation, etc.).
- Plan the database:
- Decide on the database management system (e.g., SQL Server, MySQL, etc.).
- Design the database schema (e.g., tables, relationships, etc.).
- Create a new Git repository:
- Create a new repository on a version control platform (e.g., GitHub, GitLab, etc.).
- Initialize the repository with the project files.
Deliverables:
- A new ASP.NET Core project setup with the chosen architecture.
- A database schema design document.
- A Git repository initialized with the project files.
Image
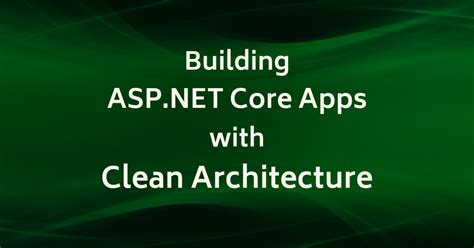
Sprint 2: Data Access and Business Logic (Estimated Time: 4-5 days)
In this sprint, we will focus on implementing the data access and business logic layers.
Tasks:
- Implement data access:
- Create a data access layer using a chosen ORM (e.g., Entity Framework Core, Dapper, etc.).
- Implement CRUD operations for the database tables.
- Implement business logic:
- Create a business logic layer to encapsulate the application's logic.
- Implement the required business rules and operations.
- Write unit tests:
- Write unit tests for the data access and business logic layers.
- Use a testing framework (e.g., xUnit, NUnit, etc.) to write and run tests.
Deliverables:
- A functional data access layer with CRUD operations.
- A business logic layer with implemented business rules and operations.
- Unit tests for the data access and business logic layers.
Image
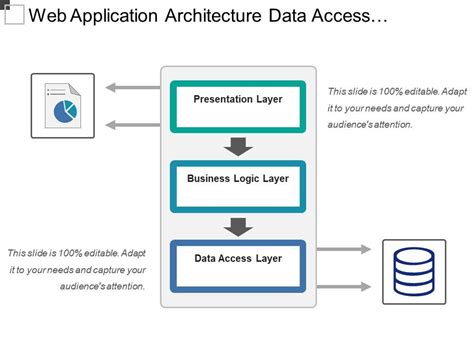
Sprint 3: Presentation Layer and API (Estimated Time: 4-5 days)
In this sprint, we will focus on implementing the presentation layer and API.
Tasks:
- Implement presentation layer:
- Choose a presentation framework (e.g., Razor Pages, MVC, etc.).
- Implement the required views and controllers.
- Implement API:
- Choose an API framework (e.g., Web API, gRPC, etc.).
- Implement the required API endpoints.
- Write integration tests:
- Write integration tests for the presentation layer and API.
- Use a testing framework (e.g., xUnit, NUnit, etc.) to write and run tests.
Deliverables:
- A functional presentation layer with views and controllers.
- A functional API with implemented endpoints.
- Integration tests for the presentation layer and API.
Image
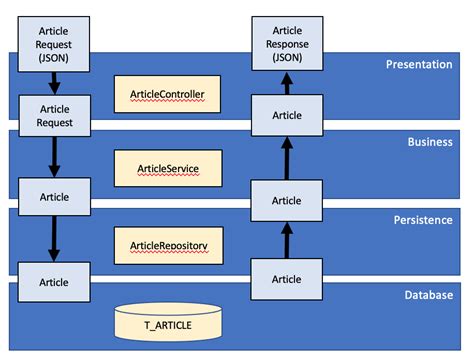
Sprint 4: Deployment and Testing (Estimated Time: 2-3 days)
In this sprint, we will focus on deploying the application and performing final testing.
Tasks:
- Deploy the application:
- Choose a deployment strategy (e.g., IIS, Docker, etc.).
- Deploy the application to a chosen environment (e.g., development, staging, production, etc.).
- Perform final testing:
- Perform manual testing of the application.
- Run automated tests (unit, integration, etc.) to ensure the application is working correctly.
- Fix any issues:
- Fix any issues found during testing.
Deliverables:
- A deployed application in a chosen environment.
- A tested application with any issues fixed.
Image

Gallery Section
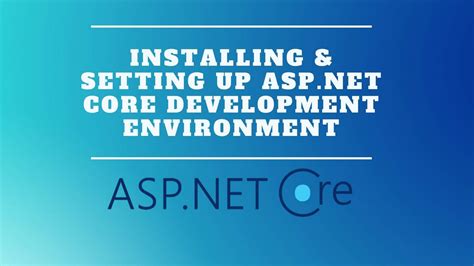
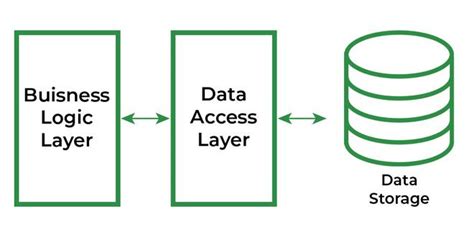

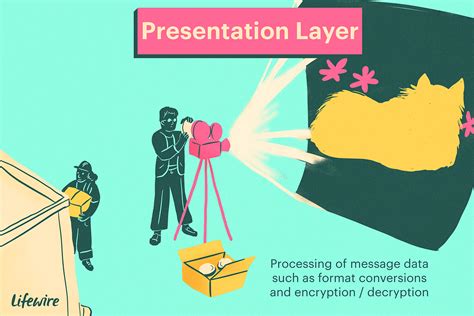


FAQ Section
What is ASP.NET Core?
+ASP.NET Core is a free, open-source, and cross-platform web framework developed by Microsoft.
What is the difference between ASP.NET Core and ASP.NET?
+ASP.NET Core is a newer, more lightweight, and flexible framework compared to ASP.NET.
What is the best way to deploy an ASP.NET Core application?
+The best way to deploy an ASP.NET Core application depends on the specific requirements and environment.