Building a project with a Go application can be a rewarding experience, especially with the right approach. Go, also known as Golang, is a statically typed, compiled language designed to be concurrent and garbage-collected, making it an ideal choice for building scalable and efficient software systems. Whether you're a seasoned developer or just starting out, here are five ways to build a project with a Go application.
1. Start with the Basics
Before diving into building a project, it's essential to have a solid grasp of the Go language fundamentals. Start by learning the basics of Go, including data types, variables, control structures, functions, and error handling. You can find plenty of resources online, including the official Go documentation, tutorials, and courses.

Once you have a good understanding of the basics, you can start exploring more advanced topics, such as concurrency, networking, and databases.
Useful Resources:
- Official Go documentation: https://golang.org/doc/
- Go by Example: https://gobyexample.com/
- Go Tour: https://tour.golang.org/
2. Choose a Project Idea
With a solid foundation in Go, it's time to choose a project idea. Consider what problems you want to solve or what interests you. You can start with simple projects, such as:
- Building a command-line tool
- Creating a RESTful API
- Developing a web scraper
- Building a chatbot
As you gain more experience, you can move on to more complex projects, such as:
- Building a distributed system
- Creating a machine learning model
- Developing a real-time analytics platform

Useful Resources:
- Go Project Ideas: https://github.com/golang/go/wiki/ProjectIdeas
- Go GitHub Repository: https://github.com/golang
3. Set Up Your Development Environment
Before starting your project, set up your development environment. You'll need:
- Go installed on your machine
- A code editor or IDE (Integrated Development Environment)
- A terminal or command prompt
- A version control system, such as Git

Useful Resources:
- Go Installation Instructions: https://golang.org/doc/install
- Go IDEs and Code Editors: https://github.com/golang/go/wiki/IDEsAndTextEditorPlugins
- Git Tutorial: https://git-scm.com/docs/gittutorial
4. Write Clean and Maintainable Code
As you start writing your project, focus on writing clean and maintainable code. Follow best practices, such as:
- Using meaningful variable names and comments
- Organizing your code into logical packages and files
- Using Go's built-in testing and debugging tools
- Following Go's coding standards and conventions

Useful Resources:
- Go Coding Standards: https://golang.org/wiki/CodeReviewComments
- Go Testing and Debugging: https://golang.org/pkg/testing/
- Go Best Practices: https://github.com/golang/go/wiki/BestPractices
5. Join the Go Community
Finally, join the Go community to connect with other developers, get help, and learn from others. You can:
- Participate in online forums and discussion groups
- Attend Go meetups and conferences
- Contribute to open-source Go projects
- Share your own projects and experiences with others
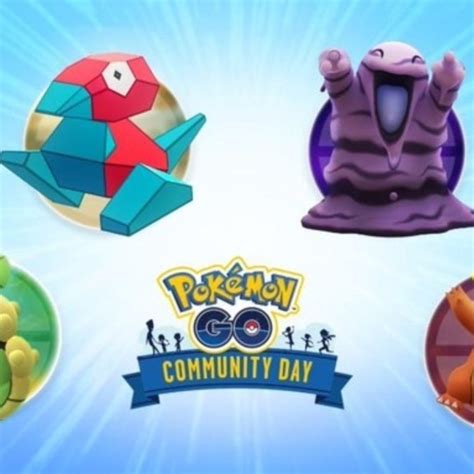
Useful Resources:
- Go Forum: https://forum.golangbridge.org/
- Go Meetups: https://www.meetup.com/topics/go/
- Go Conferences: https://github.com/golang/go/wiki/Conferences
- Go GitHub Repository: https://github.com/golang
Gallery of Go Development
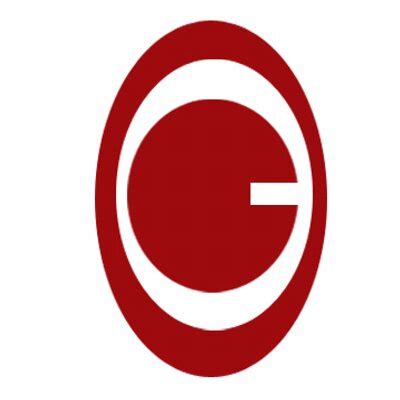

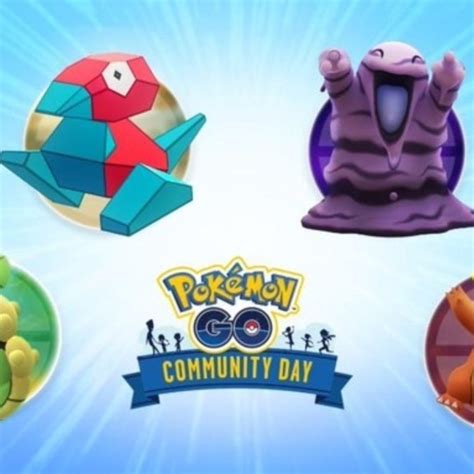


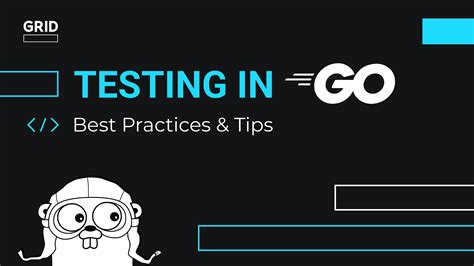
FAQ Section
What is Go?
+Go, also known as Golang, is a statically typed, compiled language designed to be concurrent and garbage-collected.
What are the benefits of using Go?
+Go is designed to be concurrent, garbage-collected, and efficient, making it an ideal choice for building scalable and efficient software systems.
How do I get started with Go?
+Start by learning the basics of Go, including data types, variables, control structures, functions, and error handling. You can find plenty of resources online, including the official Go documentation, tutorials, and courses.